This lesson teaches you to
- Provide Details for a Step
- Create and Handle User Actions
- Group Guided Steps Into a Guided Sequence
- Customize Step Presentation
Try it out
Your application might have multi-step tasks for users. For example, your app might need to guide users through purchasing additional content, or setting up a complex configuration setting, or simply confirming a decision. All of these tasks require walking users through one or more ordered steps or decisions.
The v17 Leanback support library
provides classes to implement multi-step user tasks. This lesson discusses how to use the
GuidedStepFragment
class to guide a user through a series
of decisions to accomplish a task. GuidedStepFragment
uses
TV UI best practices to make multi-step tasks easy to understand and navigate on TV devices.
Provide Details for a Step
A GuidedStepFragment
represents a single step in a series
of steps. Visually it provides a guidance view on the left with step information. On the right,
GuidedStepFragment
provides a view containing a
list of possible actions or decisions for this step.

Figure 1. An example guided step.
For each step in your multi-step task, extend
GuidedStepFragment
and provide context information about
the step and actions the user can take. Override
onCreateGuidance()
and return a new
GuidanceStylist.Guidance
that contains context
information, such as the step title, description, and icon.
@Override public GuidanceStylist.Guidance onCreateGuidance(Bundle savedInstanceState) { String title = getString(R.string.guidedstep_first_title); String breadcrumb = getString(R.string.guidedstep_first_breadcrumb); String description = getString(R.string.guidedstep_first_description); Drawable icon = getActivity().getDrawable(R.drawable.guidedstep_main_icon_1); return new GuidanceStylist.Guidance(title, description, breadcrumb, icon); }
Add your GuidedStepFragment
subclass to your desired
activity by calling
GuidedStepFragment.add()
in your activity’s onCreate()
method.
If your activity contains only GuidedStepFragment
objects, use GuidedStepFragment.addAsRoot()
instead of
add()
to add the first
GuidedStepFragment
. Using
addAsRoot()
ensures that if the user presses the Back button on the TV remote when viewing
the first GuidedStepFragment
, both the
GuidedStepFragment
and the parent activity will close.
Note: Add
GuidedStepFragment
objects programmatically
and not in your layout XML files.
Create and Handle User Actions
Add user actions by overriding
onCreateActions()
.
In your override, add a new GuidedAction
for each
action item, and provide the action string, description, and ID. Use
GuidedAction.Builder
to add new actions.
@Override public void onCreateActions(List<GuidedAction> actions, Bundle savedInstanceState) { // Add "Continue" user action for this step actions.add(new GuidedAction.Builder() .id(CONTINUE) .title(getString(R.string.guidedstep_continue)) .description(getString(R.string.guidedstep_letsdoit)) .hasNext(true) .build()); ...
Actions aren't limited to single-line selections. Here are additional types of actions you can create:
-
Add an information label action by setting
infoOnly(true)
. If you setinfoOnly
to true, the user can't select the action. To provide additional information about user choices, use label actions. -
Add an editable text action by setting
editable(true)
. Ifeditable
is true, when the action is selected the user can enter text using the remote or a connected keyboard. OverrideonGuidedActionEdited()
oronGuidedActionEditedAndProceed()
to get the modified text the user entered. -
Add a set of actions that behave as checkable radio buttons by using
checkSetId()
with a common ID value to group actions into a set. All actions in the same list with the same check-set ID are considered linked. When the user selects one of the actions within that set, that action becomes checked, while all other actions become unchecked. -
Add a date-picker action by using
GuidedDatePickerAction.Builder
instead ofGuidedAction.Builder
inonCreateActions()
. OverrideonGuidedActionEdited()
oronGuidedActionEditedAndProceed()
to get the modified date value the user entered. - Add an action that uses subactions to let the user pick from an extended list of choices. Subactions are described in Add subactions.
- Add a button action that appears to the right of the actions list and is easily accessible. Button actions are described in Add button actions.
You can also add a visual indicator—to indicate that selecting the action
leads to a new step—by setting
hasNext(true)
.
For all the different attributes that you can set, see
GuidedAction
.
To respond to actions, override
onGuidedActionClicked()
and process the passed-in
GuidedAction
. Identify the selected action by
examining GuidedAction.getId()
.
Add subactions
Some actions might require giving the user an additional set of choices. A
GuidedAction
can specify a list of
subactions that get displayed as a drop-down list of child actions.
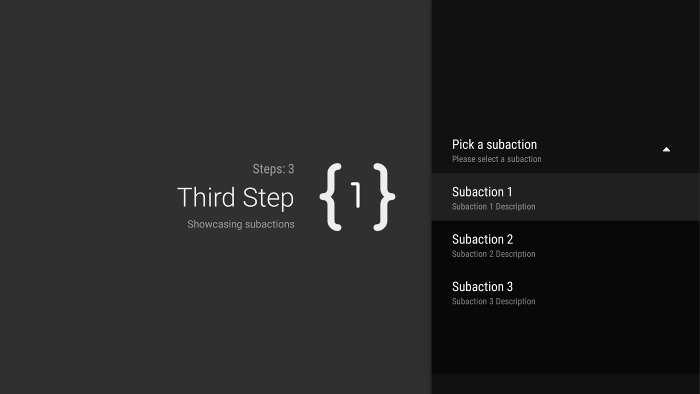
Figure 2. Guided step subactions.
The subaction list can contain regular actions or radio button actions, but not date-picker or editable text actions. Also, a subaction cannot have its own set of subactions as the system does not support more than one level of subactions. Deeply nested sets of actions create a poor user experience.
To add subactions, first create and populate a list of
GuidedActions
that will
act as subactions:
List<GuidedAction> subActions = new ArrayList<GuidedAction>(); subActions.add(new GuidedAction.Builder() .id(SUBACTION1) .title(getString(R.string.guidedstep_subaction1_title)) .description(getString(R.string.guidedstep_subaction1_desc)) .build()); ...
In onCreateActions()
, create a top-level
GuidedAction
that will display the
list of subactions when selected:
@Override public void onCreateActions(List<GuidedAction> actions, Bundle savedInstanceState) { ... actions.add(new GuidedAction.Builder() .id(SUBACTIONS) .title(getString(R.string.guidedstep_subactions_title)) .description(getString(R.string.guidedstep_subactions_desc)) .subActions(subActions) .build()); ... }
Finally, respond to subaction selections by overriding
onSubGuidedActionClicked()
:
@Override public boolean onSubGuidedActionClicked(GuidedAction action) { // Check for which action was clicked, and handle as needed if (action.getId() == SUBACTION1) { // Subaction 1 selected } // Return true to collapse the subactions drop-down list, or // false to keep the drop-down list expanded. return true; }
Add button actions
If your guided step has a large list of actions, users may have to scroll through the list to access the most commonly used actions. Use button actions to separate commonly used actions from the action list. Button actions appear to the right of the action list and are easy to navigate to.
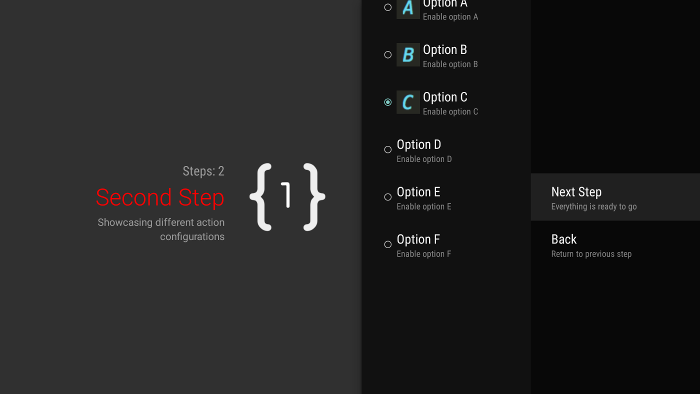
Figure 3. Guided step button actions.
Button actions are created and handled just like regular actions, but you create
button actions in
onCreateButtonActions()
instead of
onCreateActions()
. Respond to button actions in
onGuidedActionClicked()
.
Use button actions for simple actions, such as navigation actions between steps. Don't use the date-picker action or other editable actions as button actions. Also, button actions cannot have subactions.
Group Guided Steps Into a Guided Sequence
A GuidedStepFragment
represents a single step, however
you might have several steps in an ordered sequence. Group multiple
GuidedStepFragment
objects together by using
GuidedStepFragment.add()
to add
the next step in the sequence to the fragment stack.
@Override public void onGuidedActionClicked(GuidedAction action) { FragmentManager fm = getFragmentManager(); if (action.getId() == CONTINUE) { GuidedStepFragment.add(fm, new SecondStepFragment()); } ...
If the user presses the Back button on the TV remote, the device shows the previous
GuidedStepFragment
on the fragment stack. If you
decide to provide your own GuidedAction
that
returns to the previous step, you can implement the Back behavior by calling
getFragmentManager().popBackStack()
.
If you need to return the user to an even earlier step in the sequence, use
popBackStackToGuidedStepFragment()
to return to a specific
GuidedStepFragment
in the fragment stack.
When the user has finished the last step in the sequence, use
finishGuidedStepFragments()
to remove all
GuidedStepFragments
from the current stack and return to the original parent activity. If the
first GuidedStepFragment
was added
using addAsRoot()
, calling
finishGuidedStepFragments()
will also close the parent activity.
Customize Step Presentation
The GuidedStepFragment
class can use custom
themes that control presentation aspects such as title text formatting or step transition
animations. Custom themes must inherit from
Theme_Leanback_GuidedStep
, and can provide
overriding values for attributes defined in
GuidanceStylist
and
GuidedActionsStylist
.
To apply a custom theme to your GuidedStepFragment, do one of the following:
-
Apply the theme to the parent activity by setting the
android:theme
attribute to the activity element in the Android manifest. Setting this attribute applies the theme to all child views and is the easiest way to apply a custom theme if the parent activity contains onlyGuidedStepFragment
objects. -
If your activity already uses a custom theme and you don’t want to apply
GuidedStepFragment
styles to other views in the activity, add theLeanbackGuidedStepTheme_guidedStepTheme
attribute to your existing custom activity theme. This attribute points to the custom theme that only theGuidedStepFragment
objects in your activity use. -
If you use
GuidedStepFragment
objects in different activities that are part of the same overall multi-step task and want to use a consistent visual theme across all steps, overrideGuidedStepFragment.onProvideTheme()
and return your custom theme.
For more information on how to add styles and themes, see Styles and Themes.
The GuidedStepFragment
class uses special
stylist classes to access and apply theme attributes.
The GuidanceStylist
class uses theme information
to control presentation of the left guidance view, while the
GuidedActionsStylist
class uses theme information
to control presentation of the right actions view.
To customize the visual style of your steps beyond what theme customization can provide, subclass
GuidanceStylist
or
GuidedActionsStylist
and return your subclass in
GuidedStepFragment.onCreateGuidanceStylist()
or
GuidedStepFragment.onCreateActionsStylist()
.
For details on what you can customize in these subclasses, see the documentation on
GuidanceStylist
and
GuidedActionsStylist
.